There’s something comforting about it. The way it feeds back into your anxiousness, and you end up a shivering mass pressed tight to the furthest corner of the room. There’s nothing quite like it, but maybe there could be.
There was a 35 hour power outage a few weeks ago — who has too many candles now???
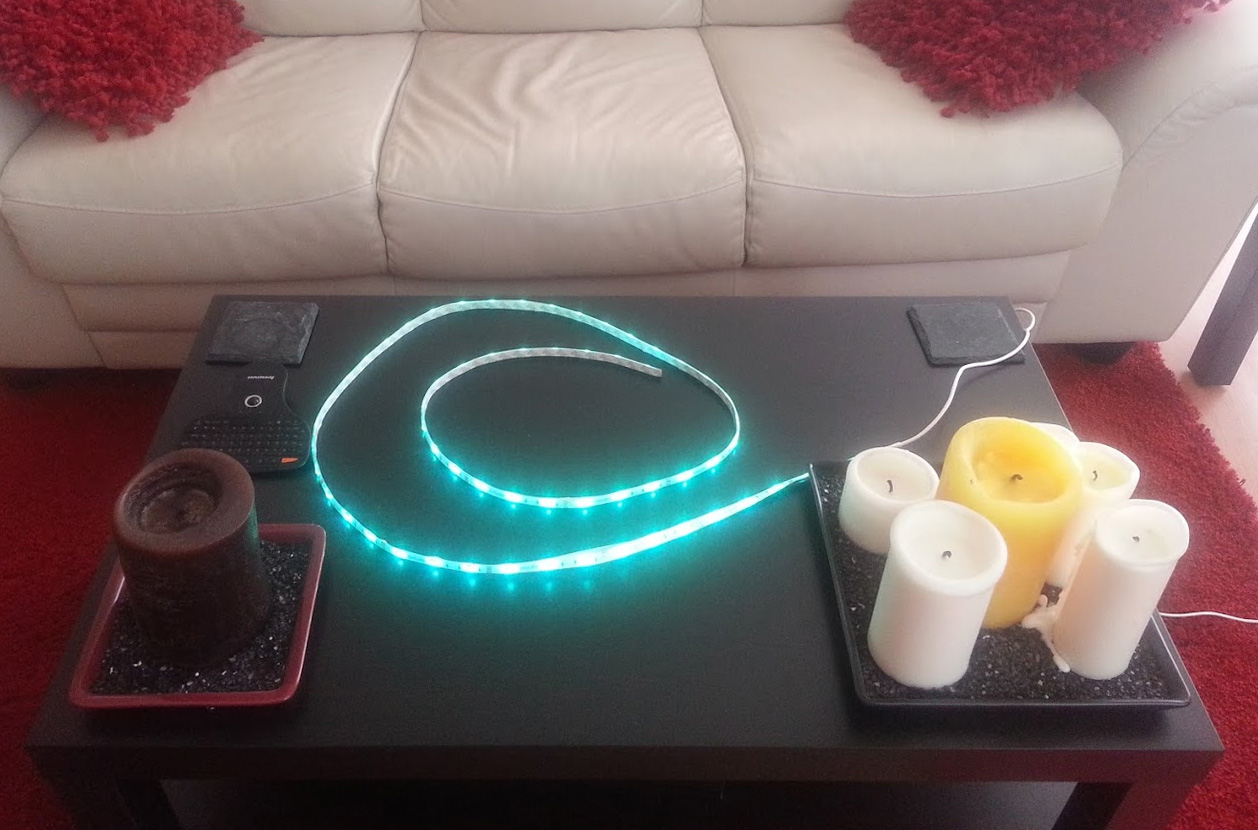
Right before that power outage, I bought some Hue LightStrips (which some may take that as a sign, but I’m nothing if not oblivious). The LightStrips are really neat and the Hue bridge comes with an an API to play with.
Using Haxe (so as to compile to various targets) I made a program to set them all aflicker, and here’s some of the code I used.
Retrieving the list of lights
public function lights() {
var output = new haxe.io.BytesOutput();
var r = new Http(api + 'lights');
r.onError = function(err) {
trace('error: ' + err);
}
r.customRequest(false, output, 'GET');
return Json.parse(output.getBytes().toString());
}
Where api is a string with the value “http://[ip-of-the-bridge]/[you-user-hash]/”. This is the sort of object you’ll receive:
{
1 => {
name => LightStrips 1,
type => Color light,
swversion => 66013452,
uniqueid => 00:17:88:01:00:cc:72:da-0b,
modelid => LST001,
state => {
effect => none,
reachable => true,
on => true,
xy => [0.1678,0.3852],
bri => 41,
hue => 35925,
sat => 254,
alert => none,
colormode => hs
},
pointsymbol => {
1 => none,
2 => none,
3 => none,
4 => none,
5 => none,
6 => none,
7 => none,
8 => none
},
manufacturername => Philips
},
2 => {
name => LightStrips 2,
type => Color light,
swversion => 66013452,
uniqueid => 00:17:88:01:00:cc:72:de-0b,
modelid => LST001,
state => {
effect => none,
reachable => true,
on => true,
xy => [0.1678,0.3852],
bri => 41,
hue => 35925,
sat => 254,
alert => none,
colormode => hs
},
pointsymbol => {
1 => none,
2 => none,
3 => none,
4 => none,
5 => none,
6 => none,
7 => none,
8 => none
},
manufacturername => Philips
}
}
The most important things here are the light keys (1, and 2), and a few of their state fields (bri, hue, sat).
Updating a light
public function update(light, point, data) {
var output = new haxe.io.BytesOutput();
var r = new Http(api + 'lights/' + light + '/' + point);
r.onError = function(err) {
trace('error: ' + err);
}
r = r.setPostData(data);
r.customRequest(false, output, 'PUT');
}
Which I call like so:
var data = {
"bri": 120,
"hue": 3000,
"sat": 100,
"transitiontime": 2 // 2*100ms
}
var ds = Json.stringify(data);
hue.update('1', 'state', ds);